dynamic reports
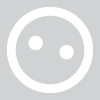
Hello,
I have an app with graphical objects (rectangles, lines, circles, textes). I need to print/export this graphic. The output should have an defined header and footer. For this I defined templates (frx files with defined page header and pages footer).
Workflow:
if user click on print button, an data file (also an frx file with shapes, lines, etc. ) will be written. After this step, a window appers where user select the requiered template, page Size, page Orientation etc. After confirming this dialog the two files will be merged with the parameters user has selected. All this steps are going fine. But sometimes the graphic is that huge, that all the objects that are placed horizontaly can't be places on page (pagesize is selected by user e.g. A4. So the graphic objects should be brocken into several pages.
Please point me to the right direction.
Thanks for any replies.
I have an app with graphical objects (rectangles, lines, circles, textes). I need to print/export this graphic. The output should have an defined header and footer. For this I defined templates (frx files with defined page header and pages footer).
Workflow:
if user click on print button, an data file (also an frx file with shapes, lines, etc. ) will be written. After this step, a window appers where user select the requiered template, page Size, page Orientation etc. After confirming this dialog the two files will be merged with the parameters user has selected. All this steps are going fine. But sometimes the graphic is that huge, that all the objects that are placed horizontaly can't be places on page (pagesize is selected by user e.g. A4. So the graphic objects should be brocken into several pages.
Please point me to the right direction.
Thanks for any replies.
Comments
- Export the content to a image
- Slice the exported image into defined pieces
- create pages in the output report (amount of pages = amount of image pieces)
- place every image-piece on depending page
<!--fonto:Courier New--><span style="font-family:Courier New"><!--/fonto-->here is the code:
...
ReportPage targetPg, currentPg;
DataBand targetBand, currentBand;
ImageExport export;
MemoryStream memStream, memSnippet;
SizeF imgSize;
Bitmap imgContent, imgSnippet;
Graphics graphic;
PictureObject imgPic;
int width, height;
bool pgExists;
export = new ImageExport();
export.ImageFormat = ImageExportFormat.Png;
export.ShowProgress = true;
memStream = new MemoryStream();
sourceReport.Prepare();
sourceReport.Export(export, memStream);
imgContent = new Bitmap(memStream);
imgSize = imgContent.PhysicalDimension;
if (imgSize.Width > targetArea.Width)
{
width = Convert.ToInt32(targetArea.Width);
height = Convert.ToInt32(imgSize.Height);
}
else if (imgSize.Height > targetArea.Height)
{
width = Convert.ToInt32(imgSize.Width);
height = Convert.ToInt32(targetArea.Height);
}
else
{
width = Convert.ToInt32(imgSize.Width);
height = Convert.ToInt32(imgSize.Height);
}
imgSnippet = new Bitmap(width, height);
graphic = Graphics.FromImage(imgSnippet);
graphic.InterpolationMode = InterpolationMode.HighQualityBicubic;
graphic.SmoothingMode = SmoothingMode.HighQuality;
graphic.PixelOffsetMode = PixelOffsetMode.HighQuality;
graphic.CompositingQuality = CompositingQuality.HighQuality;
targetPg = targetReport.Pages.OfType<ReportPage>().ToList().FirstOrDefault();
targetBand = targetPg.Bands.OfType<DataBand>().ToList().FirstOrDefault();
currentPg = targetPg;
currentBand = targetBand;
for (int x = 0; x < imgSize.Width; x += width)
{
for (int y = 0; y < imgSize.Height; y += height)
{
graphic.Clear(Color.White);
graphic.DrawImage(imgContent, new Rectangle(0, 0, width, height), x, y, width-10, height-10, GraphicsUnit.Pixel);
graphic.Flush();
memSnippet = new MemoryStream();
imgSnippet.Save(memSnippet, ImageFormat.Tiff);
memStream.Flush();
imgPic = new PictureObject();
imgPic.Name = "picContent1";
imgPic.Left = targetArea.Left;
imgPic.Top = targetArea.Top;
imgPic.Height = imgSnippet.Height;
imgPic.Width = imgSnippet.Width;
imgPic.Image = Image.FromStream(memSnippet, true);
currentBand.AddChild(imgPic);
currentBand.RemoveChild(targetArea);
pgExists = targetReport.Pages.OfType<ReportPage>().ToList().Exists(
delegate(ReportPage page) { return page.Name == currentPg.Name; });
if (pgExists == false)
{
targetReport.AddChild(currentPg);
}
currentPg = new ReportPage();
currentPg.Name = string.Format("Page{0}", targetReport.Pages.OfType<ReportPage>().ToList().Count + 1);
currentPg.AssignAll(targetPg);
currentBand = currentPg.Bands.OfType<DataBand>().ToList().FirstOrDefault();
}
}
memStream.Close();
...<!--fontc--></span><!--/fontc-->
here is the correct version:
ReportPage targetPg, currentPg;
DataBand targetBand, currentBand;
ImageExport export;
MemoryStream memStream, memSnippet;
SizeF imgSize;
Bitmap imgContent, imgSnippet;
Graphics graphic;
PictureObject imgPic;
int width, height;
bool pgExists;
export = new ImageExport();
export.ImageFormat = ImageExportFormat.Tiff;
export.ShowProgress = true;
memStream = new MemoryStream();
sourceReport.Prepare();
sourceReport.Export(export, memStream);
imgContent = new Bitmap(memStream);
imgSize = imgContent.PhysicalDimension;
if (imgSize.Width > targetArea.Width)
width = Convert.ToInt32(targetArea.Width);
else
width = Convert.ToInt32(imgSize.Width);
if (imgSize.Height > targetArea.Height)
height = Convert.ToInt32(targetArea.Height);
else
height = Convert.ToInt32(imgSize.Height);
imgSnippet = new Bitmap(width, height);
graphic = Graphics.FromImage(imgSnippet);
graphic.InterpolationMode = InterpolationMode.HighQualityBicubic;
graphic.SmoothingMode = SmoothingMode.HighQuality;
graphic.PixelOffsetMode = PixelOffsetMode.HighQuality;
graphic.CompositingQuality = CompositingQuality.HighQuality;
targetPg = targetReport.Pages.OfType<ReportPage>().FirstOrDefault();
targetBand = targetPg.Bands.OfType<DataBand>().FirstOrDefault();
currentPg = targetPg;
currentBand = targetBand;
for (int x = 0; x < imgSize.Width; x += width)
{
for (int y = 0; y < imgSize.Height; y += height)
{
graphic.Clear(Color.White);
graphic.DrawImage(imgContent, new Rectangle(0, 0, width, height), x, y, width-10, height-10, GraphicsUnit.Pixel);
graphic.Flush();
memSnippet = new MemoryStream();
imgSnippet.Save(memSnippet, ImageFormat.Tiff);
memStream.Flush();
imgPic = new PictureObject();
imgPic.Name = "picContent1";
imgPic.Left = targetArea.Left;
imgPic.Top = targetArea.Top;
imgPic.Height = imgSnippet.Height;
imgPic.Width = imgSnippet.Width;
imgPic.Image = Image.FromStream(memSnippet, true);
currentBand.AddChild(imgPic);
currentBand.RemoveChild(targetArea);
pgExists = targetReport.Pages.OfType<ReportPage>().ToList().Exists(
delegate(ReportPage page) { return page.Name == currentPg.Name; });
if (pgExists == false)
{
targetReport.AddChild(currentPg);
}
currentPg = new ReportPage();
currentPg.Name = string.Format("Page{0}", targetReport.Pages.OfType<ReportPage>().ToList().Count + 1);
currentPg.AssignAll(targetPg);
currentBand = currentPg.Bands.OfType<DataBand>().FirstOrDefault();
}
}
memStream.Close();
Modify the PaperWidth/PaperHeight properties of the report page so all the objects can fit on the page. Then use extended printing mode which splits a big page into several A4 pages:
http://www.fast-report.com/documentation/U...printreport.htm